What is Reactive Programming?
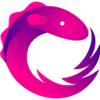
Reactive programming is the programming model needed to build reactive systems.
Asynchronous message-based communication, one of the design principles of reactive systems mentioned in the previous post, means non-blocking I/O as opposed to blocking I/O.
Blocking I/O means that any remaining work is blocked and waits until that thread finishes its work, which means that tasks waiting behind it must allocate additional threads to do their work without waiting, which is more expensive.
Non-blocking I/O, on the other hand, literally means that threads never get blocked. For this reason, it can be argued that reactive programming is a programming model that fits well with the design principles of reactive systems.
Reactive Programming Features
Reactive programming in computing represents a declarative programming approach that handles data streams and change propagation.
Since the bolded sections demonstrate the fundamental features of reactive programming we will analyze them individually.
declarative programming
Traditional programming uses imperative style as seen in C and Java which requires users to define specific actions for execution.
Declarative programming differs from imperative programming because we don’t list specific actions to perform but simply state what needs to be done.
Imperative programming instructs the system about the specific steps needed to complete a task.
Imperative programming example:
List<Integer> numberList = Arrays.asList(10, 20, 30, 45, 52, 61); int sum = 0; for(int number : numberList){ if(number > 30 && (number % 2 != 0)){ sum += number; } } System.out.println("total: " + sum);
This example demonstrates a straightforward instance of imperative programming.
This example demonstrates explicit specification of necessary operations directly within the written code.
declarative programming example:
List<Integer> numberList = Arrays.asList(10, 20, 30, 45, 52, 61); int sum = numberList.stream() .filter(number -> number > 30 && (number % 2 != 0)) .mapToInt(number -> number) .sum(); System.out.println("total: " + sum);
This example demonstrates Java Stream utilization which follows declarative programming principles while lacking reactive programming capabilities.
This example demonstrates how declarative programming avoids explicitly detailing the execution flow within the code itself.
Declarative programming centers around defining what the desired result should be rather than how to achieve it.
In other words, rather than specifying the sequential execution flow, the system determines the optimal way to execute the task internally.
data streams and the propagation of change
Data streams refer to the ongoing creation of data while “the propagation of change” defines each data creation as an event which triggers continuous data delivery during its occurrence.
Understanding the Basic Structure of Reactive Programming
Reactive programming code mainly consists of Publisher components, Subscriber components, Data Sources and Operators. Now we will examine the purpose of each component for a short duration.
Publisher
The Publisher’s role is to supply the incoming data.
Subscriber
A Subscriber receives and uses the data provided by the Publisher. In a similar context, it is also referred to as a Consumer, from the perspective of consuming data. Therefore, Subscriber and Consumer can be considered similar in meaning, as both are responsible for using the data received from the Publisher.
Data Source
A Data Source refers to the data that is delivered as input to the Publisher. Similarly, the term Data Stream is sometimes used to describe this concept.
In a nutshell, a
Data Source
can be considered the original data itself, while aData Stream
refers to the form of data that enters as input to the Publisher.
Operator
It is rare for the data delivered by the Publisher to be passed to the Subscriber without any processing. Appropriate transformations are applied between the Publisher and Subscriber according to the application’s requirements, and it is the Operator that handles this processing.
In fact, it wouldn’t be an exaggeration to say that reactive programming begins and ends with Operators. From Operators that generate data to those that handle filtering and transformation, reactive programming offers a wide variety of Operators.
What’s next?
Do you have a general understanding of reactive programming now?
In the next post, we’ll take a look at Reactive Streams.